TCP is a Connection-Oriented Protocol. The Communication can be done using Sockets. The System Calls used in the interaction of Client Server are in the library <sys/socket.h>
1) int socket(int family, int type, int protocol);
creates an entry in object/file descriptor table to allow I/O based on file descriptors and also returns the index in object description table.
2) int bind(int sockfd, const struct sockaddr *myaddr, socklen_t addrlen);
determines the local address and local process. It assigns a name to an unnamed socket. It informs system with its address that are dispatched to the process.
3) int listen(int sockfd, int backlog);
sets queue length for client process waiting to connect to socket.
4) int accept(int sockfd, const struct sockaddr *clientaddr, socklen_t addrlen);
waits for another process to connect to this socket after creating file descriptor for actual communication with client to read & write data.
5) int connect(int sockfd, const struct sockaddr *servaddr, socklen_t addrlen);
client tries to connect to waiting server process through
socket created.
6) size_t read(int filedes, void *buff, size_t nbytes);
7) size_t write(int filedes, void *buff, size_t nbytes);
8) int close(int sockfd);
CONSTANTS :
family
AF_INET IPv4 protocols
AF_INET6 IPv6 protocols
AF_LOCAL UNIX domain protocols
AF_ROUTE Routing protocols
AF_KEY Key protocols
type
SOCK_STREAM stream socket
SOCK_DGRAM datagram socket
SOCK_SEQPACKET sequenced packet socket
SOCK_RAW raw socket
protocol
IPROTO_TCP TCP transport protocol
IPROTO_UDP UDP transport protocol
IPROTO_SCTP SCTP transport protocol
CLIENT SERVER Communication Flow
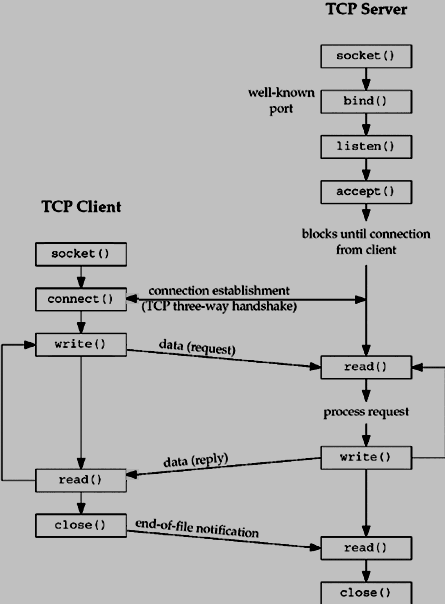
server.c
#include <stdio.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
void error(char *msg)
{
perror(msg);
exit(1);
}
int main(int argc, char *argv[])
{
int sockfd, newsockfd, portno, clilen;
char buffer[256];
struct sockaddr_in serv_addr, cli_addr;
int n;
if (argc<2)
{
fprintf(stderr,"ERROR, no port provided\n");
exit(1);
}
sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd<0)
error("ERROR opening socket");
bzero((char *) &serv_addr, sizeof(serv_addr));
portno = atoi(argv[1]);
serv_addr.sin_family = AF_INET;
serv_addr.sin_addr.s_addr = INADDR_ANY;
serv_addr.sin_port = htons(portno);
if (bind(sockfd, (struct sockaddr *) &serv_addr,
sizeof(serv_addr))<0)
error("ERROR on binding");
listen(sockfd,5);
clilen = sizeof(cli_addr);
newsockfd = accept(sockfd,(struct sockaddr *) &cli_addr,
&clilen);
if (newsockfd<0)
error("ERROR on accept");
bzero(buffer,256);
n = read(newsockfd,buffer,255);
if (n<0)
error("ERROR reading from socket");
printf("Here is the message: %s\n",buffer);
n = write(newsockfd,"SERVER : I got your message",18);
if (n<0)
error("ERROR writing to socket");
return 0;
}
client.c
#include <stdio.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <netdb.h>
void error(char *msg)
{
perror(msg);
exit(1);
}
int main(int argc, char *argv[])
{
int sockfd, portno;
char buffer[256];
struct sockaddr_in serv_addr;
struct hostent *server;
int n;
if (argc<3)
{
fprintf(stderr,"ERROR, usage %s hostname port\n",argv[0]);
exit(0);
}
portno = atoi(argv[2]);
sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd<0)
error("ERROR opening socket");
server = gethostbyname(argv[1]);
if (server == NULL)
{
fprintf(stderr,"ERROR, no such host\n");
exit(0);
}
bzero((char *) &serv_addr, sizeof(serv_addr));
portno = atoi(argv[1]);
serv_addr.sin_family = AF_INET;
bcopy((char *)server->h_addr,
(char *)&serv_addr.sin_addr.s_addr,
server->h_length);
serv_addr.sin_port = htons(portno);
if (connect(sockfd, (struct sockaddr *) &serv_addr,
sizeof(serv_addr))<0)
printf("Please Enter the message");
bzero(buffer,256);
fgets(buffer,255,stdin);
n = write(sockfd,buffer,255);
if (n<0)
error("ERROR writing to socket");
bzero(buffer,256);
n = read(sockfd,buffer,255);
if (n<0)
error("ERROR reading to socket");
printf("CLIENT : Here is the message: %s\n",buffer);
return 0;
}
OUTPUT :
$ ./server server 51222
$ ./client client manoj 51222
Please enter the message
you are going to be killed!
SERVER : I got the message
CLIENT : Here is the message: you are going to be killed!